JavaScript Base64 and URL Encoding Decoding Example
In this short article, we’ll explore JavaScript encoding decoding capabilities. In JavaScript, these are the functions respectively for encoding and decoding Base64 strings and URL.
btoa()
: This function uses the A-Za-z0-9+/= characters to encode the string in Base64.atob()
: It decodes a Base64 encoded string created bybtoa()
.encodeURI()
: ThisencodeURI()
function is used to encode a URI.decodeURI()
: It decodes a Uniform Resource Identifier (URI) previously created byencodeURI()
or by a similar routine.
Alternatively, we can also use the
encodeURIComponent(uriToEncode)
anddecodeURIComponent(encodedURI)
function to encode and decode the URI respectively.
1. JavaScript btoa()
Syntax
var encodedString = window.btoa(stringToEncode);
Parameters
stringToEncode– The binary string to encode.
Return
Base64 representation of stringToEncode.
Exceptions
InvalidCharacterError
– The string contained a character that did not fit in a single byte.
Example
var originalString = "Love the way you lie";
var encodedString = window.btoa(originalString);
console.log(encodedString);
Output
TG92ZSB0aGUgd2F5IHlvdSBsaWU=
2. JavaScript atob()
Syntax
var decodedString = window.atob(encodedString);
Parameters
encodedString– Encoded string generated by btoa()
.
Throws
DOMException
if encodedString is not valid Base64.
Example
var encodedString = "TG92ZSB0aGUgd2F5IHlvdSBsaWU=";
var decodedString = window.atob(encodedString);
console.log(decodedString);
Output
Love the way you lie
3. JavaScript encodeURI()
Syntax
var encodedURL = window.encodeURI(uriToEncode);
Parameter
uriToEncode– A complete URI.
Return
A new string representing the provided string encoded as a URI.
Example
var originalURL = "https://websparrow.org/?menu=шеллы";
var encodedURL = window.encodeURI(originalURL);
console.log(encodedURL);
Output
https://websparrow.org/?menu=%D1%88%D0%B5%D0%BB%D0%BB%D1%8B
4. JavaScript decodeURI()
Syntax
var decodedURL = window.decodeURI(encodedURL);
Parameter
endcodedURL– Encoded URI string generated by endcodedURI()
function.
Return
A new string representing the unencoded version of the given encoded Uniform Resource Identifier (URI).
Throws
URIError
exception when encodedURI contains invalid character sequences.
Example
var encodedURL = "https://websparrow.org/?menu=%D1%88%D0%B5%D0%BB%D0%BB%D1%8B";
var decodedURL = window.decodeURI(encodedURL);
console.log(decodedURL);
Output
https://websparrow.org/?menu=шеллы
Alternatively, we can also use the
encodeURIComponent(uriToEncode)
anddecodeURIComponent(encodedURI)
function to encode and decode the URI respectively.
Let’s see the complete example that you can directly run in your web browser.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Encoding Decoding Example</title>
<script>
function encodeDecode() {
// Simple string
var originalString = "Love the way you lie";
// Encoded string
var encodedString = window.btoa(originalString);
// Decoded string
var decodedString = window.atob(encodedString);
// Add all them to paragraph
document.getElementById("os").innerHTML = "Original String: " + originalString;
document.getElementById("es").innerHTML = "Encoded String: " + encodedString;
document.getElementById("ds").innerHTML = "Decoded String: " + decodedString;
}
</script>
<script>
function encodeDecodeURL() {
// URL string
var originalURL = "https://websparrow.org/?menu=шеллы";
// Encoded URL
var encodedURL = encodeURI(originalURL);
// Decoded URL
var decodedURL = decodeURI(encodedURL);
// Add all them to paragraph
document.getElementById("os-uri").innerHTML = "Original URL: " + originalURL;
document.getElementById("es-uri").innerHTML = "Encoded URL: " + encodedURL;
document.getElementById("ds-uri").innerHTML = "Decoded URL: " + decodedURL;
}
</script>
</head>
<body>
<h2>JavaScript Encoding Decoding Example</h2>
<strong>Basic Encoding Decoding</strong>
<button onClick="encodeDecode();">Run</button>
<div>
<p id="os" style="color:black;"></p>
<p id="es" style="color:red;"></p>
<p id="ds" style="color:green;"></p>
</div>
<hr />
<strong>URL Encoding Decoding</strong>
<button onClick="encodeDecodeURL();">Run</button>
<div>
<p id="os-uri" style="color:black;"></p>
<p id="es-uri" style="color:red;"></p>
<p id="ds-uri" style="color:green;"></p>
</div>
</body>
</html>
Output
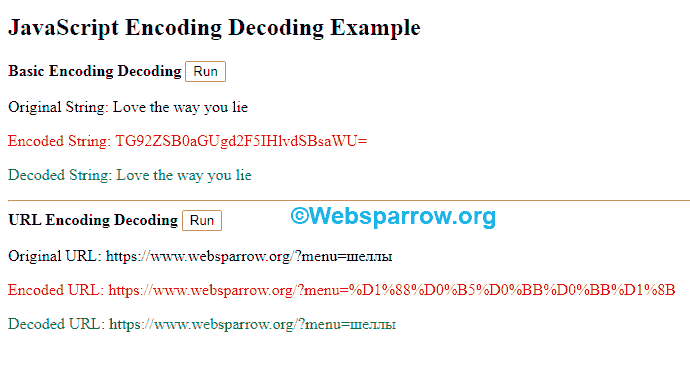